Blog
Data - How to enforce unique values (or prevent duplicate values) in one or more columns
When building apps that collect data, a common requirement is to prevent the entry of duplicate records. This post walks through how to accomplish this task by demonstrating how to enforce unique email address values in an employee table, and how to enforce a unique combination of firstname and surname for each record.
As a starting point for this post, we'll build an auto generated app that's based on this SharePoint list. As this post progresses, we'll walk through how to enforce unique email addresses, and a unique combination of firstname and surname values for each record in the list.
Best practice - Enforcing uniqueness at the data source
Whenever we want to avoid duplicates, a best practice is to enforce uniqueness at the data source level, in addition to any checks that we make in Power Apps. This prevents users from creating duplicating records outside of Power Apps.
To carry out this task in SharePoint, we select the settings of a column and click the 'enforce unqiue values' radio button.
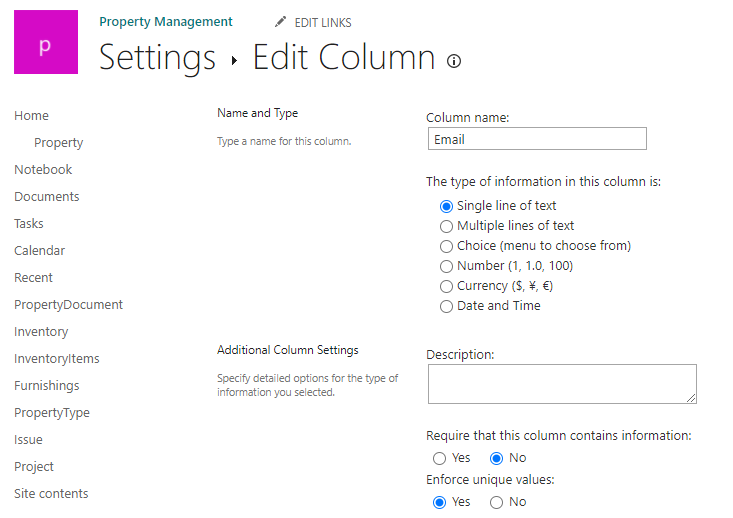
How Power Apps deals with duplicate values
When we define unique columns at the data source, Power Apps displays an error message when a user attempts to add a duplicate record.
As an example, here's the error that appears when a user attempts to create or update a record that contains a duplicate email address.
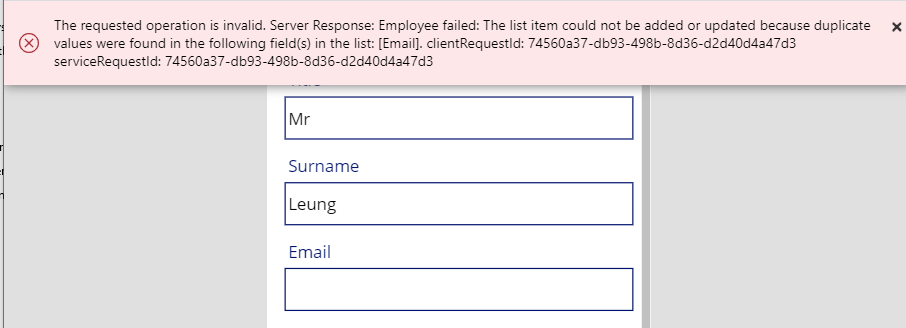
Detecting duplicate values from Power Apps when adding a record
To better handle this behaviour, we can write formula to detect the presense of a duplicate record before the call to SubmitForm (or Patch).
The formula beneath highlights the basic syntax that we can add to the submit button to detect a duplicate email address when a user attempts to create a new record.:
If(IsBlank(LookUp(Employee, Email=DataCardValue17.Text)),
SubmitForm(EditForm1),
Notify("Email must be unique",NotificationType.Error);
SetFocus(DataCardValue17)
)
Otherwise, the formula calls the Notify function to alert the user. Next, it calls the SetFocus function to set the focus to the email text input control.
The screenshot beneath highlights the notification that appears when the user enters a duplicate email address.
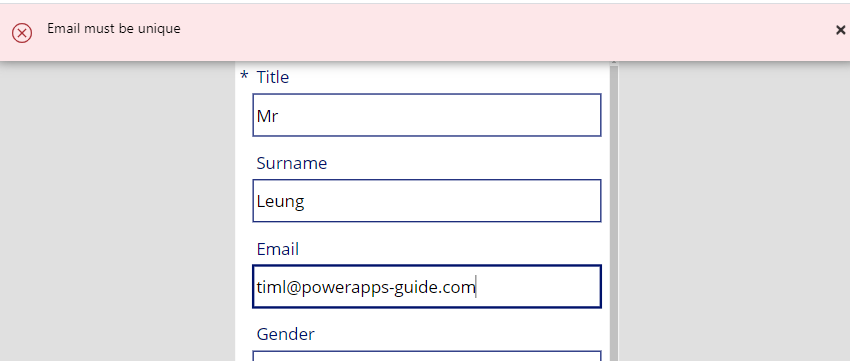
Enforcing unqiueness accross multiple columns
To enforce uniqueness across multiple columns, we can adapt our formula to reference the multiple columns in the call to LookUp. Here's the syntax to enforce a unique combination of firstname and surname for each record in the employee list.
If(IsBlank(LookUp(Employee, Firstname=DataCardValue11.Text
And Surname=DataCardValue19.Text))
,
SubmitForm(EditForm1),
Notify("Error - duplicate values detected",NotificationType.Error)
)
If(IsBlank(LookUp(Employee, Email=DataCardValue17.Text))
And
IsBlank(LookUp(Employee, Firstname=DataCardValue11.Text
And Surname=DataCardValue19.Text))
,
SubmitForm(EditForm1),
Notify("Error - duplicate values detected",NotificationType.Error)
)
Detecting duplicate values from Power Apps when creating and editing a record
With a SharePoint list, the ID field uniquely identifies each record. We can use this to identify whether any duplicate record is genuinely a duplicate, rather than the record that matches the existing record.
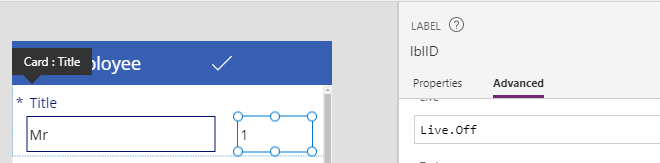
Here's the formula that carries out this logic.
With({matchingRecords:Filter(Employee, Email=EditForm1.Updates.Email Or
(Firstname = EditForm1.Updates.Firstname And
Surname = EditForm1.Updates.Surname))}
,
If(IsEmpty(matchingRecords) Or
(CountRows(matchingRecords) = 1 And
First(matchingRecords).ID = Value(lblID.Text)),
SubmitForm(EditForm1),
Notify("Error - duplicate values detected",NotificationType.Error)
)
)
Conclusion
- Categories:
- data
- duplicate records
- Data - How to remove trailing comma all rows in a table
- Data - How to find the common rows from 3 or more collections
- Data - How to show the distinct rows from 2 data sources or collections
- Data - How to implement circular rotational date sorting
- Bug - What to do when the data section of the Power Apps Maker portal doesn't work
- Data - Combine columns from separate tables into a single table
- Formula - Transposing/converting rows to columns- an almost impossible task?
- Data - How to rename field names in a record
- Data - How to hide duplicate rows in a gallery / show distinct multiple columns in a gallery
- Data - Retrieving news/forum/blog articles with RSS
- Data - How to sort by partial numbers in a text field
- Data - How to return the last record from a table
- Data - How to create bulk test/dummy records with random values
- Data - 3 things you should know before using the MySQL or PostgreSQL connectors
- Data - A walkthrough of how to migrate the data source of an app from Excel to Sharepoint
- Data - How much mobile data does Power Apps consume? What ways can we minimise this?
- Data - How to save and retrieve Google calendar entries
- Data - How to save and retrieve Google contacts
- SQL - Caution! This is how users can hack shared SQL connections
- SharePoint – 2 Mistakes to avoid when importing Excel data
- SQL - Don't let this DateTime bug catch you out!
- Settings - What's the purpose of the "Explicit Column Selection" Setting?
- SQL Server for Beginners Part 3 - Installing On-Premises Gateway
- SQL Server for Beginners Part 2 - Installing Management Studio
- SQL Server for Beginners Part 1 - Installing SQL Server
- Searching data–What you need to know about case sensitivity
- Images - How to create images that can change depending on data
- Excel - Reasons NOT to use Excel as a data source
- SharePoint - What you need to know about Filtering Data
- Formulas - Generating Row Numbers