Blog
Data - How to find the common rows from 3 or more collections
October 6. 2024
In an app with multiple collections, how can we find identical rows across three or more collections? This post describes the formula that we can use.
When working with data, there is often a need to find common rows across multiple data sources or collections.
With two data sources, the process can be relatively straightforward. However, as the number of data sources increases, the complexity of the task also increases.
This post provides a strategy to address this challenge and demonstrates the process using three collections.Example - Creating source collections
Formula to find matching records across 3 collections
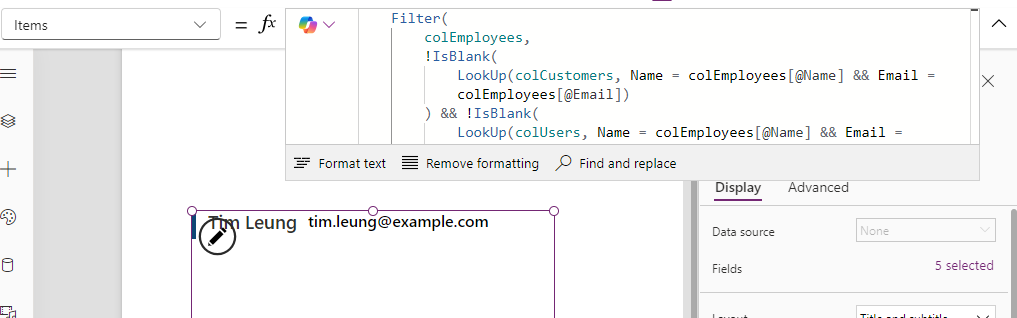
With two data sources, the process can be relatively straightforward. However, as the number of data sources increases, the complexity of the task also increases.
This post provides a strategy to address this challenge and demonstrates the process using three collections.
Example - Creating source collections
For this example, let's take the following 3 example collections - Employees, Customers, and Users.
Notice how the "Tim Leung" user appears in all 3 collections. This demonstration will build a formula to identify this user.
ClearCollect(
colEmployees,
{ Name: "Alice Johnson", Email: "alice.johnson@example.com" },
{ Name: "Bob Smith", Email: "bob.smith@example.com" },
{ Name: "Tim Leung", Email: "tim.leung@example.com" },
{ Name: "Charlie Brown", Email: "charlie.brown@example.com" }
);
ClearCollect(
colCustomers,
{ Name: "David Lee", Email: "david.lee@example.com" },
{ Name: "Tim Leung", Email: "tim.leung@example.com" },
{ Name: "Eve Adams", Email: "eve.adams@example.com" },
{ Name: "Alice Johnson", Email: "alice.johnson@example.com" }
);
ClearCollect(
colUsers,
{ Name: "Frank White", Email: "frank.white@example.com" },
{ Name: "Grace Green", Email: "grace.green@example.com" },
{ Name: "Tim Leung", Email: "tim.leung@example.com" },
{ Name: "Bob Smith", Email: "bob.smith@example.com" }
);
Formula to find matching records across 3 collections
To find records that appear in all three collections, we can use the following syntax:
Filter(
colEmployees,
!IsBlank(
LookUp(colCustomers, Name = colEmployees[@Name] && Email = colEmployees[@Email])
) &&
!IsBlank(
LookUp(colUsers, Name = colEmployees[@Name] && Email = colEmployees[@Email])
)
)
The formula takes one of the tables as a base (colEmployees).
It filters this collection and returns records where calling LookUp on the colCustomers and colUsers collections returns a non-blank value. The presence of a non-blank value means the value(s) must also exist in the colCustomers and colUsers collections.
The call to LookUp matches both the Name and Email values. We can modify this to include more or fewer columns.
To verify the result, the screenshot below illustrates how the formula returns the expected record from our source collections (the Tim Leung record).
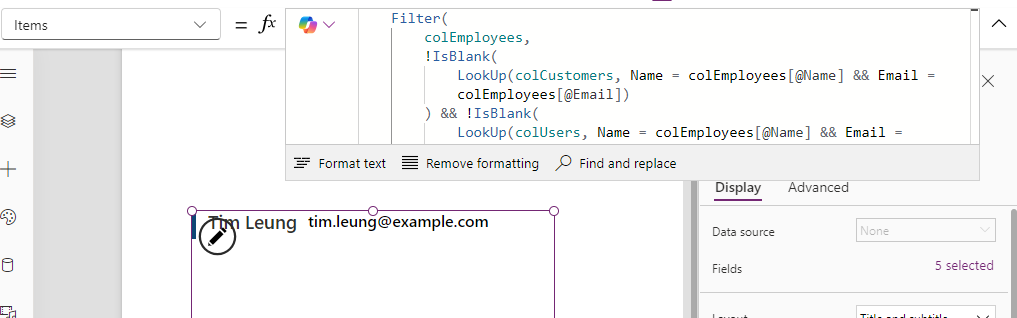
Conclusion
By using the provided strategy and formula, we can efficiently identify common records across multiple data collections. This method simplifies the process, and we can extend the calls to !IsBlank/Lookup to support a greater number of data sources.
- Categories:
- data
Related posts
- Data - How to show the distinct rows from 2 data sources or collections
- Data - How to implement circular rotational date sorting
- Bug - What to do when the data section of the Power Apps Maker portal doesn't work
- Data - Combine columns from separate tables into a single table
- Formula - Transposing/converting rows to columns- an almost impossible task?
- Data - How to rename field names in a record
- Data - How to hide duplicate rows in a gallery / show distinct multiple columns in a gallery
- Data - Retrieving news/forum/blog articles with RSS
- Data - How to sort by partial numbers in a text field
- Data - How to return the last record from a table
- Data - How to create bulk test/dummy records with random values
- Data - 3 things you should know before using the MySQL or PostgreSQL connectors
- Data - A walkthrough of how to migrate the data source of an app from Excel to Sharepoint
- Data - How to enforce unique values (or prevent duplicate values) in one or more columns
- Data - How much mobile data does Power Apps consume? What ways can we minimise this?
- Data - How to save and retrieve Google calendar entries
- Data - How to save and retrieve Google contacts
- SQL - Caution! This is how users can hack shared SQL connections
- SharePoint – 2 Mistakes to avoid when importing Excel data
- SQL - Don't let this DateTime bug catch you out!
- Settings - What's the purpose of the "Explicit Column Selection" Setting?
- SQL Server for Beginners Part 3 - Installing On-Premises Gateway
- SQL Server for Beginners Part 2 - Installing Management Studio
- SQL Server for Beginners Part 1 - Installing SQL Server
- Searching data–What you need to know about case sensitivity
- Images - How to create images that can change depending on data
- Excel - Reasons NOT to use Excel as a data source
- SharePoint - What you need to know about Filtering Data
- Formulas - Generating Row Numbers