Blog
Data - How to implement circular rotational date sorting
February 21. 2024
An interesting requirement that can arise is the requirement to implement circular sorting. This occurs typically when we want to sort date ranges based on today's date.
For example, given an input list of data, if today is Wednesday, a circular sort implementation will sort records starting with Wednesday, followed by Thursday, Friday, Saturday, Sunday, Monday, and finally Tuesday.
For reference, there are a couple of forum posts that provide more details.
How to apply circular sorting based on weekday name
To give an example, let's take a list of meeting hosts.
ClearCollect(The requirement is to show the meeting hosts for the next 7 days, starting from today's date.
colMeetingHosts,
{DayName:"Monday", MeetingHost:"John Smith"},
{DayName:"Tuesday", MeetingHost:"Mary Jones"},
{DayName:"Wednesday", MeetingHost:"Robert Lee"},
{DayName:"Thursday", MeetingHost:"Lisa Chen"},
{DayName:"Friday", MeetingHost:"James Wilson"},
{DayName:"Saturday", MeetingHost:"Sarah Green"},
{DayName:"Sunday", MeetingHost:"David Miller"}
)
With this example data, we would need to convert the day names to numbers to implement the sort. The formula would look like this.
ClearCollect(colMeetingHostsDays,
AddColumns(
colMeetingHosts,
"DayNum",
Switch(
DayName,
"Monday", 1,
"Tuesday", 2,
"Wednesday", 3,
"Thursday", 4,
"Friday", 5,
"Saturday", 6,
"Sunday", 7
)
)
)
This produces the following result:
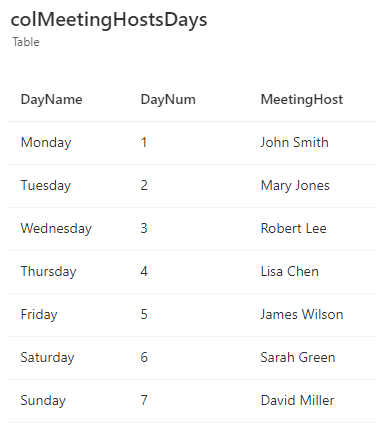
We can then sort the result like so.
Sort(
colMeetingHostsDays,If(DayNum < Weekday(Today(), StartOfWeek.Monday), "b" & Text(YourWeekNumCol), "a" & Text(YourWeekNumCol) ), SortOrder.Ascending
)
The theory here is to build a calculated 'sort' column. Let's say that today is Wednesday. We would prefix days 3 and above with 'a', and days under 3 with 'b'. We can then carry out an 'alpha' sort to return the days in the desired sequence.
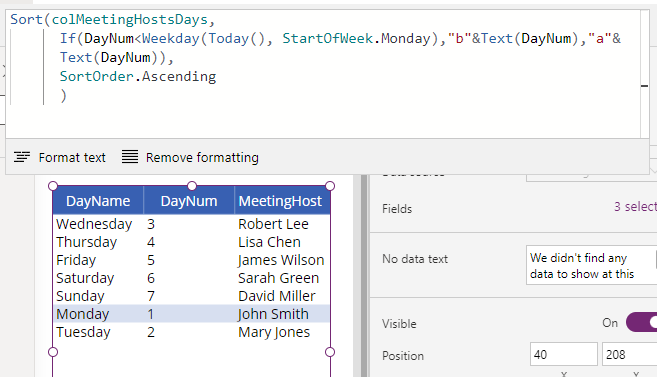
To clarify this further, this screenshot shows the calculated sort value to illustrate how the data is sorted.
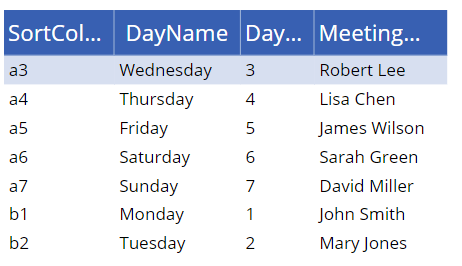
How to apply circular sorting based on month name
To give another example, here's a list of public holidays for the year.
ClearCollect(
colPublicHolidays,
{MonthName: "January", Holiday: "New Year's Day"},
{MonthName: "January", Holiday: "Martin Luther King Jr. Day"},
{MonthName: "February", Holiday: "Presidents' Day"},
{MonthName: "March", Holiday: ""},
{MonthName: "April", Holiday: "Good Friday"},
{MonthName: "April", Holiday: "Easter"},
{MonthName: "May", Holiday: "Memorial Day"},
{MonthName: "June", Holiday: ""},
{MonthName: "July", Holiday: "Independence Day"},
{MonthName: "August", Holiday: ""},
{MonthName: "September", Holiday: "Labor Day"},
{MonthName: "October", Holiday: "Columbus Day"},
{MonthName: "November", Holiday: "Veterans Day"},
{MonthName: "November", Holiday: "Thanksgiving Day"},
{MonthName: "December", Holiday: "Christmas Day"}
)
To display the public holidays for the next 12 months starting with today's month, we would use this formula.
Sort(
colPublicHolidays,
With({MonthNum:Month(DateValue("1 " & MonthName & " 2024"))},
If(MonthNum < Month(Today()),
"b" & Text(MonthNum, "00"),
"a" & Text(MonthNum, "00")
)
),
SortOrder.Ascending
)
Again, we sort by a calculated 'sort' column.
We use the technique here to convert the month names (eg January, February) to month numbers.
When we create the calculated sort column, note how we need to apply a Text format of "00". This ensures that the month numbers 1-9 are formatted as 01-09 to ensure that the sort takes account of months 10,11, and 12 correctly.
The final result looks like this based on today's month (February). We can see here how the January records appear at the end of the list.
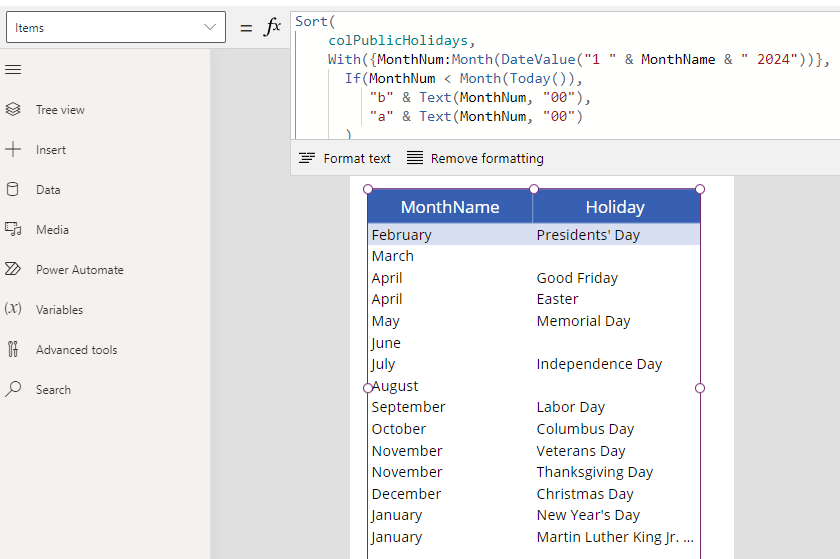
Conclusion
To implement circular sorting, we can call the Sort function and sort by a calculated expression. This post provided two examples of how to implement this type of sorting.
- Categories:
- data
Related posts
- Data - How to remove trailing comma all rows in a table
- Data - How to find the common rows from 3 or more collections
- Data - How to show the distinct rows from 2 data sources or collections
- Bug - What to do when the data section of the Power Apps Maker portal doesn't work
- Data - Combine columns from separate tables into a single table
- Formula - Transposing/converting rows to columns- an almost impossible task?
- Data - How to rename field names in a record
- Data - How to hide duplicate rows in a gallery / show distinct multiple columns in a gallery
- Data - Retrieving news/forum/blog articles with RSS
- Data - How to sort by partial numbers in a text field
- Data - How to return the last record from a table
- Data - How to create bulk test/dummy records with random values
- Data - 3 things you should know before using the MySQL or PostgreSQL connectors
- Data - A walkthrough of how to migrate the data source of an app from Excel to Sharepoint
- Data - How to enforce unique values (or prevent duplicate values) in one or more columns
- Data - How much mobile data does Power Apps consume? What ways can we minimise this?
- Data - How to save and retrieve Google calendar entries
- Data - How to save and retrieve Google contacts
- SQL - Caution! This is how users can hack shared SQL connections
- SharePoint – 2 Mistakes to avoid when importing Excel data
- SQL - Don't let this DateTime bug catch you out!
- Settings - What's the purpose of the "Explicit Column Selection" Setting?
- SQL Server for Beginners Part 3 - Installing On-Premises Gateway
- SQL Server for Beginners Part 2 - Installing Management Studio
- SQL Server for Beginners Part 1 - Installing SQL Server
- Searching data–What you need to know about case sensitivity
- Images - How to create images that can change depending on data
- Excel - Reasons NOT to use Excel as a data source
- SharePoint - What you need to know about Filtering Data
- Formulas - Generating Row Numbers