Blog
Controls - How to build a control to enter measurements in inches with fractional units
February 5. 2024
If you need to build data entry screens that permit the entry of fractional units, this post walks through how to carry out this task with the help of a slider control.
I've recently needed to build data entry input screens to enter imperial measurements and wanted a simple way to enter fractional units. I came across this great forum post here by Eric Thomas on using a slider control.
This post describes this method in a bit more detail and includes some
adaptations to enable the logic to work in European locales
that use the comma symbol as a decimal point separator.
How to create a control to enter fractional units in inches
The method that's described in the post uses a slider control. This control enables users to enter measurements in 1⁄16 inch increments. The end result looks like this.

As highlighted, a label next to the slider control shows the selected measurement using fractional units.
To build this feature, we add a slider control to our screen (named sldInches in thie code). Next to the slider, we add a label with the text property set as follows:
Concatenate(
Text(RoundDown(sldInches.Value/16, 0)),
Switch(
Mod(sldInches.Value, 16),
1, " 1/16",
2, " 1/8",
3, " 3/16",
4, " 1/4",
5, " 5/16",
6, " 3/8",
7, " 7/16",
8, " 1/2",
9, " 9/16",
10, " 5/8",
11, " 11/16",
12, " 3/4",
13, " 13/16",
14, " 7/8",
15, " 15/16"
)
)
Each unit of the slider control represents 1⁄16 of an inch. Therefore, we divide the slider value by 16 to determine the number of whole inches.
The Mod function returns the remainder which effectively gives us the fractional unit.
We call the Switch statement to return a more readable fractional value. For example, If we were to output the Mod result over 16, we would end up with half values that are represented as 8⁄16 rather than the more sensible display value of 1⁄2 .
We call the Switch statement to return a more readable fractional value. For example, If we were to output the Mod result over 16, we would end up with half values that are represented as 8⁄16 rather than the more sensible display value of 1⁄2 .
Improving the presentation of the fractional unit
To
improve the presentation of the label that displays the fraction, we
can use the HTML control to display the nominator using superscript and the denominator using subscript. The text property of the HTML control would look like this:
Concatenate(
Text(RoundDown(sldInches.Value/16, 0)),
Switch(
Mod(sldInches.Value, 16),
1, " <sup>1</sup>⁄<sub>16</sub>",
2, " <sup>1</sup>⁄<sub>8</sub>",
3, " <sup>3</sup>⁄<sub>16</sub>",
4, " <sup>1</sup>⁄<sub>4</sub>",
5, " <sup>5</sup>⁄<sub>16</sub>",
6, " <sup>3</sup>⁄<sub>8</sub>",
7, " <sup>7</sup>⁄<sub>16</sub>",
8, " <sup>1</sup>⁄<sub>2</sub>",
9, " <sup>9</sup>⁄<sub>16</sub>",
10, " <sup>5</sup>⁄<sub>8</sub>",
11, " <sup>11</sup>⁄<sub>16</sub>",
12, " <sup>3</sup>⁄<sub>4</sub>",
13, " <sup>13</sup>⁄<sub>16</sub>",
14, " <sup>7</sup>⁄<sub>8</sub>",
15, " <sup>15</sup>⁄<sub>16</sub>"
)
)
Retrieving the decimal value in inches
Typically, we want to retrieve the decimal value - particularly if we want to store the result in a data source. To do this, we divide the slider value by 16.
sldInches.Value /16
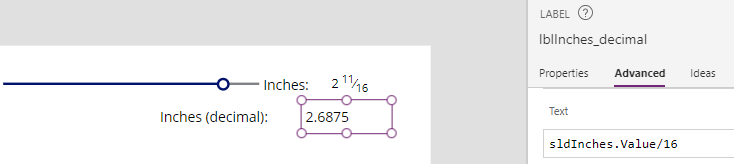
Setting the slider control with a decimal value in inches
To set the value of the slider control based on a decimal value in inches, we set the Default value to the value multiplied by 16. We can use this technique if we are setting the control based on a value from a data source.To make sure the slider control shows a valid value (in the case where the input value doesn't correspond to a 1⁄16 value), we can round down to the lower 16th value by calling RoundDown.
RoundDown(decimalValueInInches * 16 ,0)
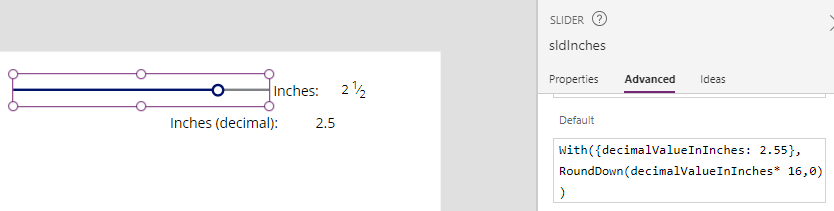
Modifying the granularity of the control
If we want to enable data entry in 1⁄8 increments rather than 1⁄16 , we would rebase our formulas to work on a value of 8 rather than 16.
The label text formula would look like this:
Retrieving the decimal value would look like this:
The label text formula would look like this:
Concatenate(
Text(RoundDown(sldInches.Value/8, 0)),
Switch(
Mod(sldInches.Value, 8),
1, " 1/8",
2, " 1/4",
3, " 3/8",
4, " 1/2",
5, " 5/8",
6, " 3/4",
7, " 7/8"
)
)
Retrieving the decimal value would look like this:
sldInches.Value /8
Finally, setting the default value of the slider would look like this:
RoundDown(decimalValueInInches * 8 ,0)
Conclusion
- Categories:
- controls
Related posts
- Controls - How to build a component for users to enter numbers with a set of connected slider and text input controls
- Controls - How to use the Tab List control - Examples
- Controls - How to set height of nested galleries dynmically
- Barcodes - How to scan barcodes - a summary of the 3 available barcode scanning controls
- Controls - How to create multi-line tooltips or multi-line text input control hint text
- Controls - How to add a clickable image / image button
- Controls - Restrict text input control to whole numbers only
- Controls - How to submit a form (or to run formula) when a user presses enter/return on the keyboard
- Controls - How to create rounded labels - workaround
- Controls - How to enter and display Office/Microsoft 365 email addresses with a combo box
- Gallery control - How to set no selected item in a gallery
- Model Driven App - How to apply an input mask to a text input field
- Controls - How to reset or clear data entry controls, and form values
- Controls - How to Transition/Show/Hide controls with a Sliding Effect
- Gallery Control - How to Paginate Data