Blog
Controls - How to set the data source of a Combo Box to a comma separated string
There may be a need to set the data source of a combo box control to a comma-separated list. This post walks through how to carry out this task.
This can occur when we want to display combo box values from a variable, custom connector, web service, or some other third-party data source.
How to set the data source of a combo box to CSV string
To demonstrate, let's take the example of a comma-separated list of days that looks like this:
"Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday"
To configure a combo box control to display each day name, we would set the items property of the combo box control like so:
Split(
"Monday,Tuesday,Wednesday,Thursday,Friday,Saturday,Sunday",
","
)
The Split function takes two input values - an input string and the separator character. It returns a single value table with the column name 'Result'. Therefore to display this output in a combo box, it's important to specify the Display Fields value - [''Result'].
In practice, source values can often include leading or trailing spaces between each item. To remove these extraneous spaces, we can call the substitute function on the input string to replace instances where there is an extra space following each comma. We would set the items property of the combo box control like so
Split(
Substitute(
"Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday",
", "
),
","
)
How to set the selected combo box items
To set the selected items in a combo box, we set the DefaultSelectedItems property. Let's assume we want to select the items Tuesday and Thursday. We would set the default selected items property of the combo box like so:
Split("Tuesday,Thursday",
","
)
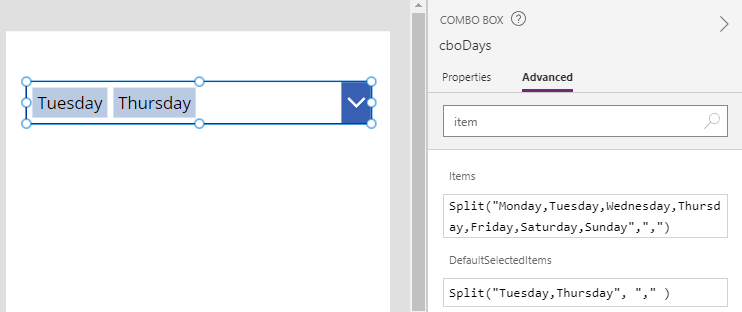
What to do when the selected combo box items don't display
As an example, let's take a variation where we set the Items property of the combo box to Calendar.WeekdaysLong. This is a built-in function that returns all the days of a week. It returns a single value table with the column name "Value".
Calendar.WeekdaysLong()
Since the return value from Split function is a table with the column name "Result", we must rename this to "Value". Here's the formula to set the values Wednesday and Friday.
RenameColumns(
Split(
"Wednesday,Friday",
","
),
"Result",
"Value"
)
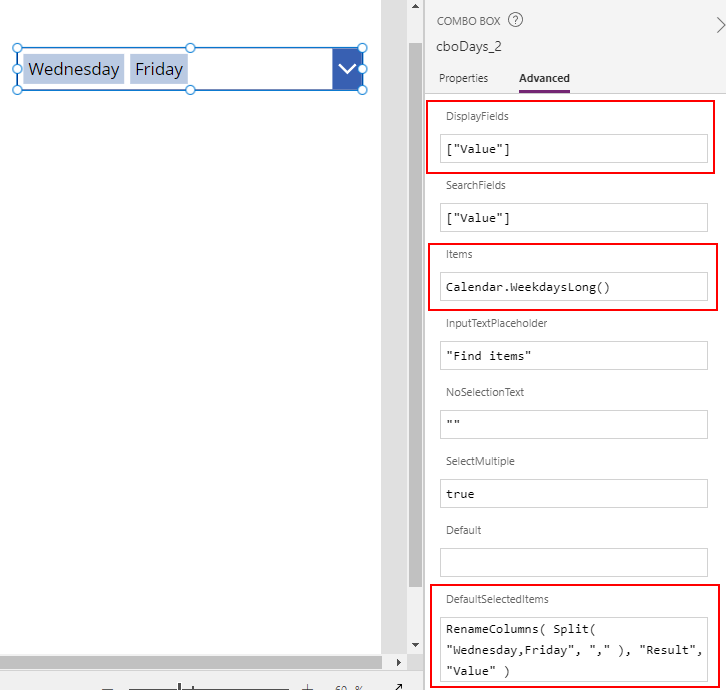
How to retrieve a comma-separated list of selected combo box items
Finally, an associated requirement to is output the selected items in a combo box control as a comma-separated string. We can accomplish this by calling the Concat function like so.
Concat(cboDays.SelectedItems, Result & ", ")
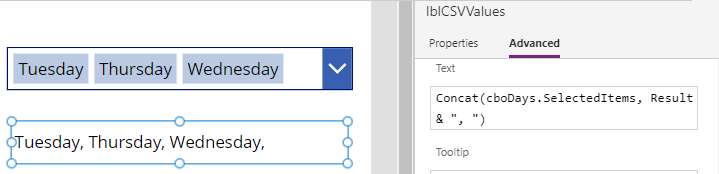
How to strip trailing commas
With({outputString:Concat(cboDays.SelectedItems, Result & ", ")},
Mid(outputString, 1, Len(outputString)-2)
)
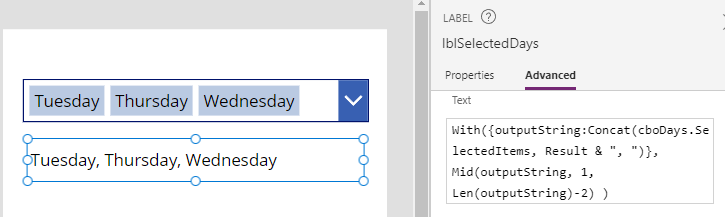
Conclusion
- Categories:
- formula
- FormuIas - Is it possible to call a user-defined function recursively in Power Apps?
- Formulas - A beginners guide on how to create and call user-defined functions (UDFs)
- Formula - How to add a button that converts degrees Centigrade to Fahrenheit and vice versa
- Formula - How to convert a single delimited string to rows and columns
- Data - How to group data in a gallery and calculate sums
- Formula - How to calculate compound interest
- Utilities - The best way to peform OCR on images of Power Apps Formulas
- Example - How to use a drop down control to convert currencies
- Formula - How to parse JSON in Power Apps- 4 examples
- Data - How to get a row by ordinal number
- Formula - What to do when the If statement doesn't work?
- Formula - Boolean And / Or operators - What is the order of precedence?
- Numbers - 10 examples of how to round numbers
- Formula - Difference between round, square, and curly brackets
- Top 3 highlights of upcoming enhancements to the Power Apps language (Power FX)
- Email - Sending email attachments with the Office 365 Outlook connector
- Formula - What to try when numbers don't format correctly
- Controls - How to convert HTML to Text
- Formulas - how to return all days between two dates
- Formula - How to create comma separated (CSV) list of items
- Formula - How to use the IF and Switch functions - 3 common examples
- Location - Finding the closest location and and sorting records by distance, based on the current location of the user
- Formulas - How to cope with weekends and public holidays in date calculations