Blog
Formulas - A beginners guide on how to create and call user-defined functions (UDFs)
If you want to improve your app by defining reusable parameterised functions, this post provides an introductory guide on how to use this new feature.
The great news is that Microsoft has started to roll out the 'user-defined function' feature. This enables us to define parameterised, reusable blocks of formula that we can call throughout an app.
How to enable the User-Defined Function Feature
To begin using this feature, the first step is to enable the feature in the app settings. There are two settings to enable. 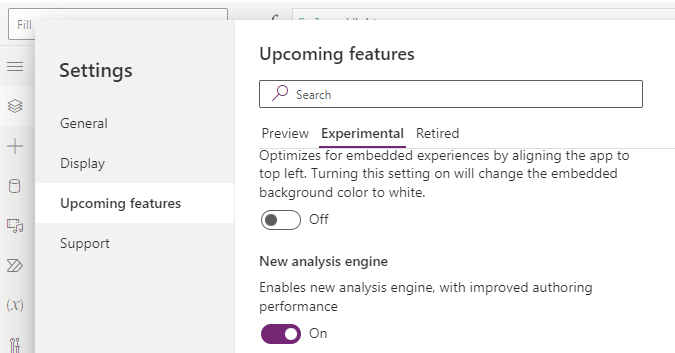
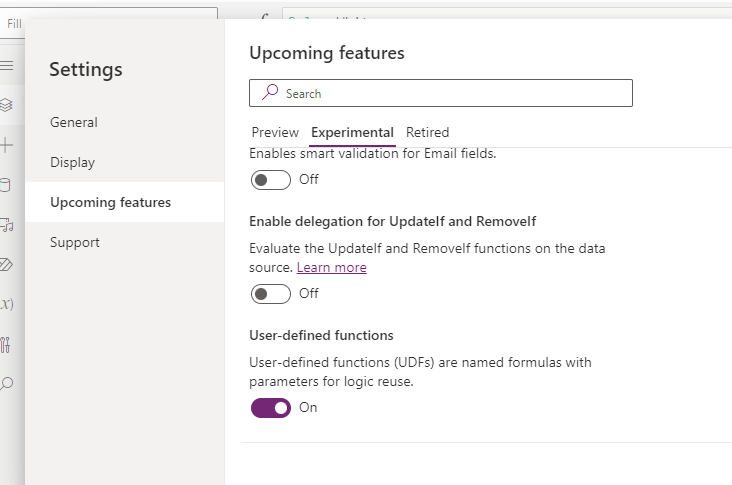
Background - User-defined functions are based on named formulas
To give some background on user-defined functions, this is a feature that builds on top of the named formulas. Named formulas are snippets of formulas that are defined
at an application level which we can call from any place in our app.
A big benefit of named formulas (amongst many others) is the deferred
execution of code. We can use this to improve performance by moving formula that traditionally runs in the OnStart of an app, to named formulas instead.
How to create a simple user-defined function
To
demonstrate a simple user-defined function, let's implement a function that converts temperatures in
Celsius to Fahrenheit. The algorithm for this function is relatively simple - we multiply the
input value by 1.8 and add 32.
To create this user-defined function, click the app node in the tree node and enter the following function definition.
CelciusToF (input:Number): Number = (input * 1.8) + 32;
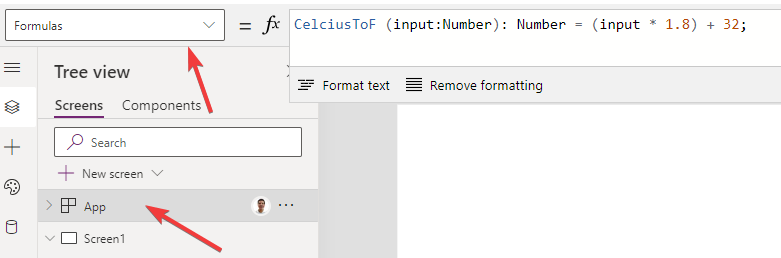
We can now reference this formula from within our app. In the example below, we can add a slider control (Slider1) that enables a user to enter a temperature in Celsius. We can then add a label that displays the Fahrenheit equivalent by using the following formula.
CelciusToF (Slider1.Value)
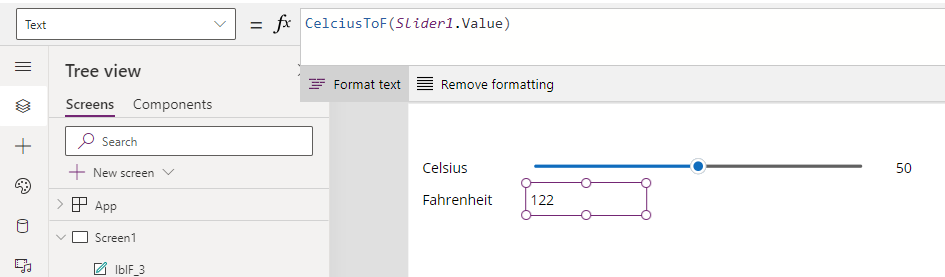
What's the syntax for defining a user-defined function?
Let's
take a closer look at our user-defined formula syntax. The named formula definition begins
with the name. Inside the brackets, we define zero or more parameters with their corresponding data types. The parameter name and data type are separated with a colon.
Following the input parameters, we enter a colon and
specify the output data type. The formula that defines the logic appears after the equals sign.What data types are supported by user-defined functions?
At the moment, user-defined functions accept the following input and output data types:- Boolean
- Color
- Date
- DateTime
- GUID
- Hyperlink
- Number
- Text
- Time
- UntypedObject
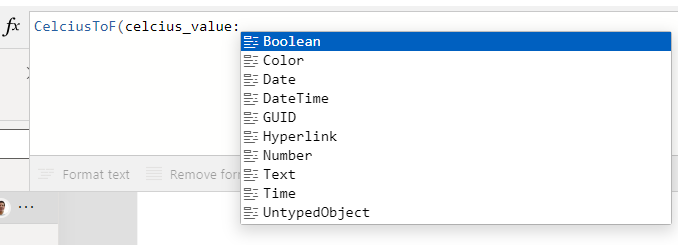
The notable data types that are not yet supported include image, record, and table.
Creating a user-defined function with multiple parameters
For another example, here's how to implement a user-defined function
to add working days to an input date. This demonstrates how to create a user-defined function
with two input parameters.
AddWorkingDays (startDate:Date, daysToAdd:Number): Date =
DateAdd(startDate
,) +
daysToAdd
RoundDown(/5, 0)*2 +
daysToAdd
Switch(Weekday(startDate
, StartOfWeek.Monday),
5,If(Mod(, 5)>0,2,0),
daysToAdd
4,If(Mod(, 5)>1,2,0),
daysToAdd
3,If(Mod(, 5)>2,2,0),
daysToAdd
2,If(Mod(, 5)>3,2,0),
daysToAdd
1,If(Mod(, 5)>4,2,0)
daysToAdd
);
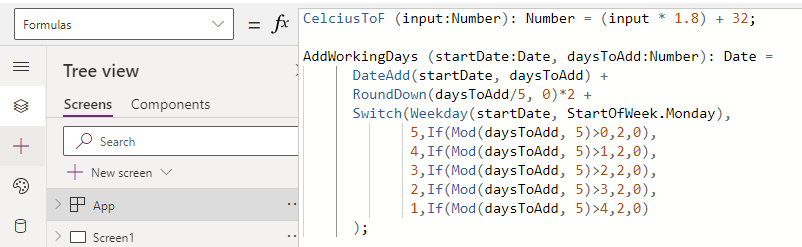
AddWorkingDays(Today(), 3)
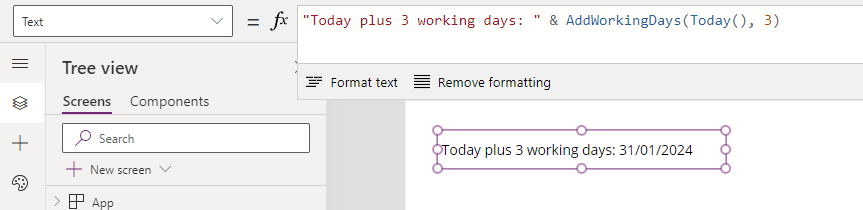
Calling user-defined functions from user-defined functions
FormatDateDDMMYY(inputDate:Date):Text = Text(inputDate, "dd/mm/yy");
AddWorkingDaysText (startDate:Date, daysToAdd:Number): Text =
FormatDateDDMMYY(DateAdd(startDate, daysToAdd) +
RoundDown(daysToAdd/5, 0)*2 +
Switch(Weekday(startDate, StartOfWeek.Monday),
5,If(Mod(daysToAdd, 5)>0,2,0),
4,If(Mod(daysToAdd, 5)>1,2,0),
3,If(Mod(daysToAdd, 5)>2,2,0),
2,If(Mod(daysToAdd, 5)>3,2,0),
1,If(Mod(daysToAdd, 5)>4,2,0)
)
);
Creating user-defined functions that connect to a data source
GetEmployeeNameByID(id:Text):Text =Although it's possible to retrieve data, it's not possible to update data in a data source. If we attempt to call functions that include Patch, Update, or Remove, we receive the error "Behavior function in a non-behavior property. You can't use this property to change values elsewhere in the app."
LookUp(Employees, id=EmployeeID, Firstname & " " & Surname);

Conclusion
- Categories:
- formula
- FormuIas - Is it possible to call a user-defined function recursively in Power Apps?
- Formula - How to add a button that converts degrees Centigrade to Fahrenheit and vice versa
- Formula - How to convert a single delimited string to rows and columns
- Data - How to group data in a gallery and calculate sums
- Formula - How to calculate compound interest
- Utilities - The best way to peform OCR on images of Power Apps Formulas
- Example - How to use a drop down control to convert currencies
- Formula - How to parse JSON in Power Apps- 4 examples
- Data - How to get a row by ordinal number
- Formula - What to do when the If statement doesn't work?
- Formula - Boolean And / Or operators - What is the order of precedence?
- Controls - How to set the data source of a Combo Box to a comma separated string
- Numbers - 10 examples of how to round numbers
- Formula - Difference between round, square, and curly brackets
- Top 3 highlights of upcoming enhancements to the Power Apps language (Power FX)
- Email - Sending email attachments with the Office 365 Outlook connector
- Formula - What to try when numbers don't format correctly
- Controls - How to convert HTML to Text
- Formulas - how to return all days between two dates
- Formula - How to create comma separated (CSV) list of items
- Formula - How to use the IF and Switch functions - 3 common examples
- Location - Finding the closest location and and sorting records by distance, based on the current location of the user
- Formulas - How to cope with weekends and public holidays in date calculations