Blog
How to split the URL parameter name and values from a hyperlink
If you need to parse hyperlinks and retrieve parameter values, this post describes how to carry out this task.
How to return a table of parameter names and values for a hyperlink
For this demonstration, let's assume that our hyperlink value is in a text input control called txtURL. The content of this text input control contains the following URL:
https://www.mywebsite.net/Search?customerFirstName=John&customerLastName=Doe&address1=88&city=New%20York&postalCode=412&country=GB
We can use the syntax below to return a table of the name-value pairs of parameters and arguments. This formula retrieves the content after the '?' by calling the Split function. It then calls the Split function with the '&' character to return a single-column table that contains the name/value parameter pairs. The formula then calls ForAll to iterate through this result and to Split the parameter name and values by the '=' character.
ForAll(Split(Last(Split(txtURL.Text,"?")).Value,
"&"),
With({param:Split(Value, "=")},
{Name:First(param).Value, Value:Last(param).Value}
)
)
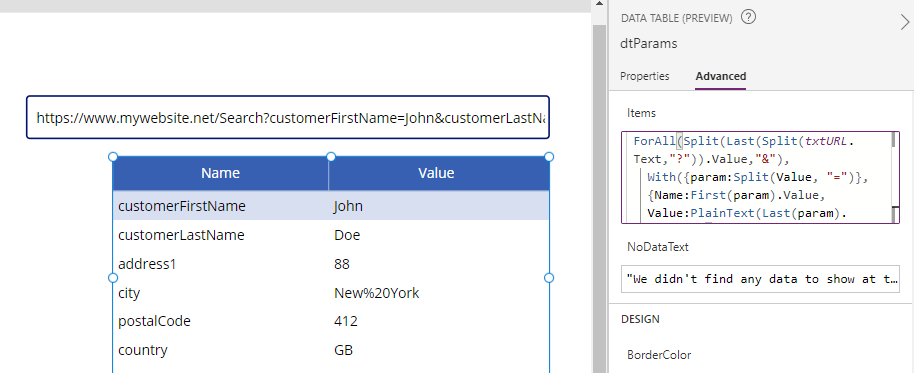
How to retrieve a hyperlink parameter value by name
To retrieve a specific parameter value, the easiest way is to extract the name-value pairs to a collection by following the formula.
ClearCollect(colParams,
ForAll(Split(Last(Split(txtURL.Text,"?")).Value,
"&"),
With({param:Split(Value, "=")},
{Name:First(param).Value, Value:Last(param).Value}
)
)
)
We can then return a specific parameter value by calling the LookUp function like so:
LookUp(colParams, Name="city").Value
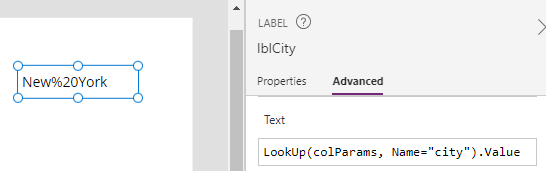
Substitute(LookUp(colParams, Name="city").Value,
"%20",
" "
)
Conclusion
- Categories:
- text
- Text - How to truncate and add 3 dots / ellipsis to long label text
- Numbers - How to Format Numbers with a Dollar Symbol the Right Way
- Text - How to extract email addresses that are formatted with angle brackets
- Text - The easiest way to copy text to the clipboard
- Text - How to split input strings by carriage return followed by the colon character
- Text - How to convert a character to its ASCII numeric value
- Why doesn't the BeginWith, EndsWith, and Contains operators work as expected?