Blog
Why doesn't the BeginWith, EndsWith, and Contains operators work as expected?
June 20. 2021
The code completion suggestions in the formula bar can be confusing. In particular, the usage of the BeginsWith, EndsWith, and Contains keywords are not entirely clear, and some app builders struggle to understand how to carry out the required string comparisons. This post describes this behaviour, including the correct syntax to carry out these operations.
The code completion suggestions that IntelliSense offers can be confusing. The most common questions I see relate to the string comparison functions contains and begins with.
As an example, let's take the example of a data entry form for entering issues. If the issue description contains the word 'child', the requirement is to show a warning that prompts the user to carry out risk assessment. This example use case scenario is to provide the means to safeguard vulnerable people.
To build this feature, the app builder adds a warning label to a screen, and starts to build the formula to set the Visible property of the label. The app builder starts a conditional statement by typing an 'If' statement, and notices that the formula bar suggests the keyword "Contains". This seems like the perfect keyword to use, and the app builder then attempts to construct a formula using 'contains', but inevitably fails. The same thing also applies with the BeginsWith keyword.
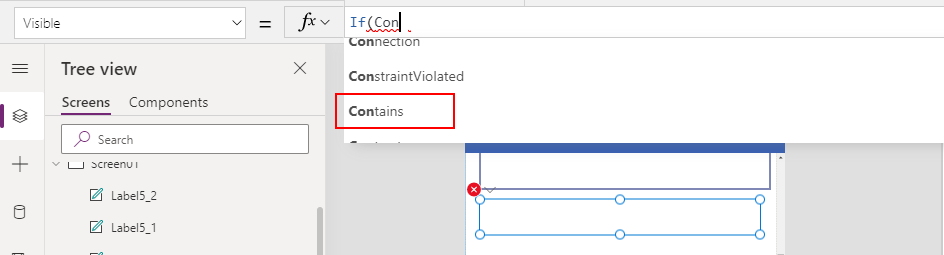
Why do the Contains and BeginsWith operators not work?
The reason why the Contains and EndsWith suggestions don't work as expected, is because they are not operators. They are enumeration values for use with the IsMatch function.The official documentation (shown beneath), highlights these three keywords.
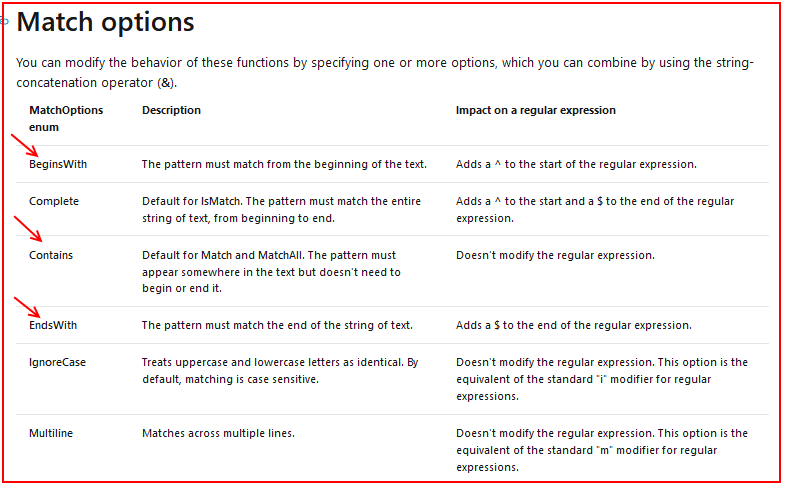
One thing that is confusing is that EndsWith is actually a valid function, in addition to being a MatchOptions enumeration value.
How to correctly use the Contains and BeginsWith operators
//Returns true if the text input control contains "child"
IsMatch(txtIssueDesc.Text, "child",
MatchOptions.Contains
)
//Returns true if the text input control begins with "child"
IsMatch(txtIssueDesc.Text, "child",
MatchOptions.BeginsWith
)
//Returns true if the text input control ends with "child"
IsMatch(txtIssueDesc.Text, "child",
MatchOptions.EndsWith
)
Note that the match process is case sensitive by default. We can enforce case insensitive matches by appending the IgnoreCase option with the & operator like so:
//Returns true if the text input control contains "child"
IsMatch(txtIssueDesc.Text, "child",
MatchOptions.Contains & MatchOptions.IgnoreCase
)
//Returns true if the text input control begins with "child"
IsMatch(txtIssueDesc.Text, "child",
MatchOptions.BeginsWith & MatchOptions.IgnoreCase
)
//Returns true if the text input control ends with "child"
IsMatch(txtIssueDesc.Text, "child",
MatchOptions.EndsWith & MatchOptions.IgnoreCase
)
Alternative formula
The alternative way to carry out these same tasks is to use the 'in, StartsWith, and EndsWith functions like so://Returns true if the text input control contains "child"
("child" in txtIssueDesc.Text)
//Returns true if the text input control begins with "child"
StartsWith(txtIssueDesc.Text, "child")
//Returns true if the text input control ends with "child"
EndsWith(txtIssueDesc.Text, "child")
Conclusion
When carrying out text comparison operations, the code suggestions in the formula bar can be confusing. Specifically, 'contains' is not a function, and the correct way to check if a piece of text starts with a specified value is to call StartsWith, and not BeginsWith.- Categories:
- text
Related posts
- Text - How to truncate and add 3 dots / ellipsis to long label text
- How to split the URL parameter name and values from a hyperlink
- Numbers - How to Format Numbers with a Dollar Symbol the Right Way
- Text - How to extract email addresses that are formatted with angle brackets
- Text - The easiest way to copy text to the clipboard
- Text - How to split input strings by carriage return followed by the colon character
- Text - How to convert a character to its ASCII numeric value