Blog
Email - Complete guide to validating email addresses
June 2. 2021
This post provides a guide on how to validate email address, including how to validate single and multiple email addresses, and how to accept or to reject email addresses based on email domain.
When we build data entry screens that capture email addresses, it's important to verify that the input addresses are valid. This post highlights several typical requirements that are related to email validation.
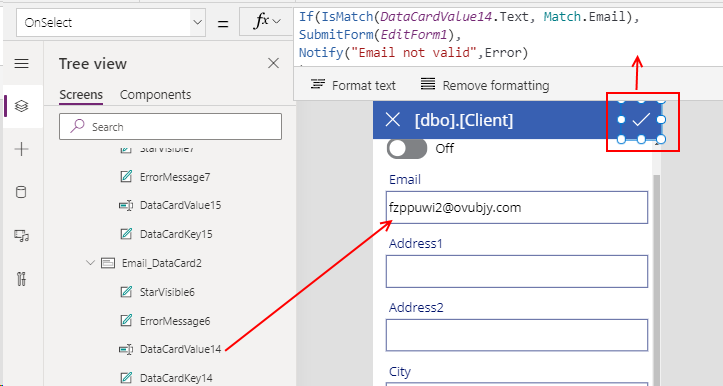
Why NOT to use the built-in Match.Email pattern with IsMatch
1. How to validate a single email address
2. How to accept emails that match a whitelist of allowable domains only
A common requirement is to provide a whitelist of acceptable domains, and to accept email addresses that match the specified list only.
The formula beneath will accept email addresses that match the domains "microsoft.com" and "contoso.com" only.
3. How to reject emails that match a blacklist of unacceptable domains
Conclusion
How to validate email addresses using the IsMatch function
As a starting point, let's begin with an edit form that's taken from an auto-generated app.
A common technique to validate email addresses is to apply the IsMatch function and to specify the built-in Match.Email pattern. The offical documentation below provides full details.
As
this documentation describes, the Email pattern "matches an email
address that contains an "at" symbol ("@") and a domain name that
contains a dot (".")".
Therefore, we can validate the existance of a valid email address by attaching the following formula to the OnSelect property of the save icon. If the email address is valid, the formula submits the form. Otherwise, it calls the Notify function to display an error message.
If(IsMatch(DataCardValue14.Text, Match.Email),
SubmitForm(EditForm1),
Notify("Email not valid",NotificationType.Error)
)
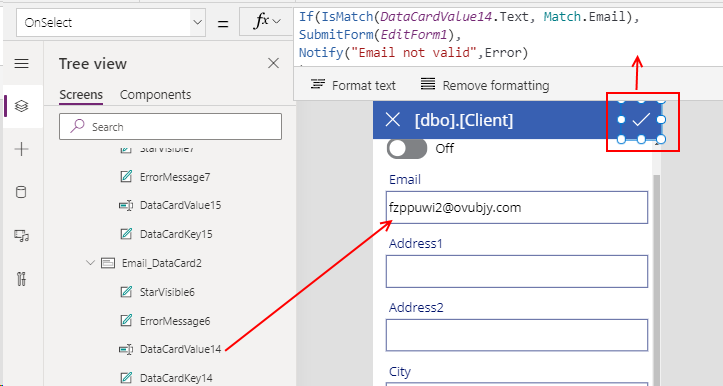
Why NOT to use the built-in Match.Email pattern with IsMatch
The reason why not to use the built-in Match.Email pattern is because it accepts email addresses that are blatantly invalid. In particular, it accepts the entry of spaces inside an email address.
If we were to apply the this IsMatch function against the following invalid email addresses with the Match.Email pattern, the result will be true, even though the email addresses are obviously not valid.
If we were to apply the this IsMatch function against the following invalid email addresses with the Match.Email pattern, the result will be true, even though the email addresses are obviously not valid.
fzppuwi2 @ ovubjy . com
fzppuwi2@ ovubjy .com,
fzppuwi2 ; @ , ovubjy . com
fzppuwi2@ovubjy.com <sfd>
1. How to validate a single email address
A more accurate way to validate a single email address is to pass a custom regular expression to the IsMatch function.
The formula beneath will rejects the invalid email addresses from the previous example, and therefore offers a more accurate method of validation.
If(IsMatch(DataCardValue14.Text,
"^([a-zA-Z0-9_.-])+@(([a-zA-Z0-9-])+.)+([a-zA-Z0-9]{2,4})+$"
),
SubmitForm(EditForm1),
Notify("Email not valid", NotificationType.Error)
)
2. How to accept emails that match a whitelist of allowable domains only
The formula beneath will accept email addresses that match the domains "microsoft.com" and "contoso.com" only.
If(IsMatch(DataCardValue14.Text,
"^([a-zA-Z0-9_.-])+@(([a-zA-Z0-9-])+.)+([a-zA-Z0-9]{2,4})+$"
) And
Last(Split(DataCardValue14.Text, "@")).Result
in ["gmail.com", "microsoft.com"]
,
SubmitForm(EditForm1),
Notify("Email not valid", NotificationType.Error)
)
3. How to reject emails that match a blacklist of unacceptable domains
A natural extension of the previous example is to reject email addresses that belong to a blacklist of domains.
The formula beneath rejects email addresses that match the domains "mailinator.com", "yopmail.com", and "maildrop.cc" (eg, temporary/disposable email services).4 Validate multiple email addresses, semi-colon separated
.
If(IsMatch(DataCardValue14.Text,
"^([a-zA-Z0-9_.-])+@(([a-zA-Z0-9-])+.)+([a-zA-Z0-9]{2,4})+$"
) And
Not(Last(Split(DataCardValue14.Text, "@")).Result
in ["gmail.com", "microsoft.com"])
,
SubmitForm(EditForm1),
Notify("Email not valid", NotificationType.Error)
)
4 Validate multiple email addresses, semi-colon separated
To validate multiple semi-colon separated email addresses, we can apply a suitable regular expression. The example beneath applies a regular expression that's taken from the following Stackoverflow post:
If(IsMatch(DataCardValue14.Text,
"(([a-zA-Z0-9_\-\.]+)@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.)|(([a-zA-Z0-9\-]+\.)+))([a-zA-Z]{2,4}|[0-9]{1,3})(\]?)(\s*;\s*|\s*$))*""
),
SubmitForm(EditForm1),
Notify("Email not valid", NotificationType.Error)
)
This pattern successfully matches the following email addresses:
abc@abc.com - validates
abc@abc.com;123@qwer.com - validates
abc@abc.com; - does not validate
empty string - validates
Conclusion
To validate the entry of a valid email address, the best practice is to call the IsMatch function and to provide a suitable regular expression. This post described how to validate single and multiple email addresses, and how to accept or reject email addresses based on email domain.
- Categories:
Previous
Related posts
- Bug - Sending email messages with the Office365Outlook connector that includes SharePoint file attachments no longer works
- Email - Sending messages with SMTP connector
- Email - How to save multiple email addresses in a field, and configure the selection and display of addresses through a combo box
- Email - How to open a new Outlook mail message and pre-populate the subject and message
- Email - How to send email without the Office 365 Outlook connector (using the Mail connector)
- Email - How to send mail with the Gmail connector