Blog
Navigation - How to build dynamic data driven navigation, or to restrict access to screens based on data
An occasional question that I hear from app builders is - how do I build dynamic, or data driven navigation structures in Power Apps? This post describes a strategy that we can use.
- Building access control features where we define the screens that each user can access in a SharePoint list (or other data source).
- Building 'dynamic navigation' where an app navigates different users to different screens, depending on data.
Demonstration - How to show a list of screens in a gallery control
To start with a simple example, here's how to display a list of screens in a gallery control.
In the OnStart property of the app, we set a variable that stores the screens along with an identifier.
Set(varMenu,
Table({Id:1, Desc:"Properties", Screen:PropertyBrowseScreen},
{Id:2, Desc:"Property Types", Screen:PropertyTypeBrowseScreen},
{Id:3, Desc:"Employees", Screen:EmployeeBrowseScreen}
)
)
Navigate(ThisItem.Screen)
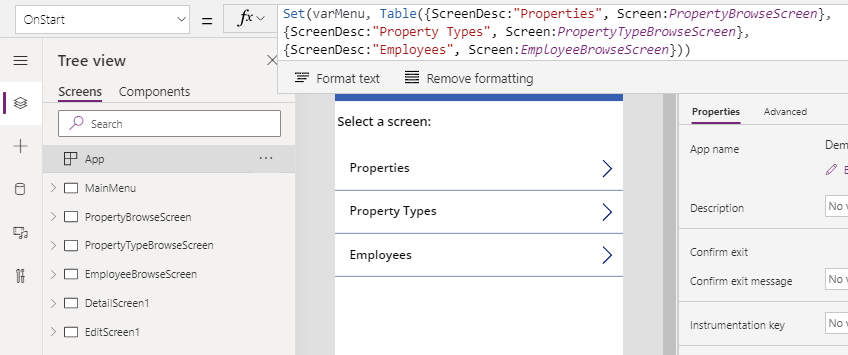
How to restrict access to screens depending on the logged on user
Extending the example above, we can now add a feature to restrict access to screens based on the email address of the logged on user.
In the OnStart property of our app, we can add the following formula to add a collection that defines the email addresses of the user that are permitted to access the screens in the app. In practice, we could store this in a SharePoint list or other data source.
ClearCollect(colAccess,
{screenId:1, email:"tim@powerapps-guide.com"},
{screenId:2, email:"tim@powerapps-guide.com"},
{screenId:3, email:"tim@powerapps-guide.com"},
{screenId:1, email:"sally@powerapps-guide.com"},
{screenId:2, email:"sally@powerapps-guide.com"}
)
AddColumns(
Filter(colAccess, email=User().Email),
"Desc",
LookUp(varMenu, Id=screenId).Desc,
"Screen",
LookUp(varMenu, Id=screenId).Screen
)
Conclusion
- Categories:
- screens